型くりこについてはこちらをご覧ください。
目次
「Gear/歯車」について
文字通り、歯車のような図形を描く技法です。






「三角関数のcos()とsin()を使用して円を描く」という方法にワンポイント加えるだけで描くことができます。歯車の目の細かさなどを調整することでいろいろなパターンの図形を描くことができます。
使い方
「三角関数のcos()とsin()を使用して円を描く」際に、半径の大きさをでこぼこさせます。
カウンターなどを用意し、円を描いていく中でカウンターの値を使って「でこ」と「ぼこ」のどちらなのかを判断することで実現できます。
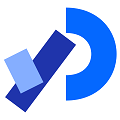
void setup() {
size(720, 720, P2D);
background(0);
int counter = 0;
beginShape();
// 6カウントを1区切りとして考えることにするので、
// PIに6の倍数(今回は24)で割った値を加えていく
for (float r = 0; r <TAU; r+= PI / 24) {
// カウンターの値に応じて半径の長さを変える
float d = 0;
if (counter % 6 >= 3) {
d = 360;
} else {
d = 240;
}
vertex(cos(r) * d + width / 2, sin(r) * d + height / 2);
counter++; // カウンターを増やすのを忘れない
}
endShape();
}
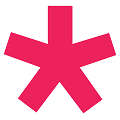
function setup() {
createCanvas(720, 720);
background(0);
var counter = 0;
beginShape();
// 6カウントを1区切りとして考えることにするので、
// PIに6の倍数(今回は24)で割った値を加えていく
for (var r = 0; r <TAU; r+= PI / 24) {
// カウンターの値に応じて半径の長さを変える
var d = 0;
if (counter % 6 >= 3) {
d = 360;
} else {
d = 240;
}
vertex(cos(r) * d + width / 2, sin(r) * d + height / 2);
counter++; // カウンターを増やすのを忘れない
}
endShape();
}
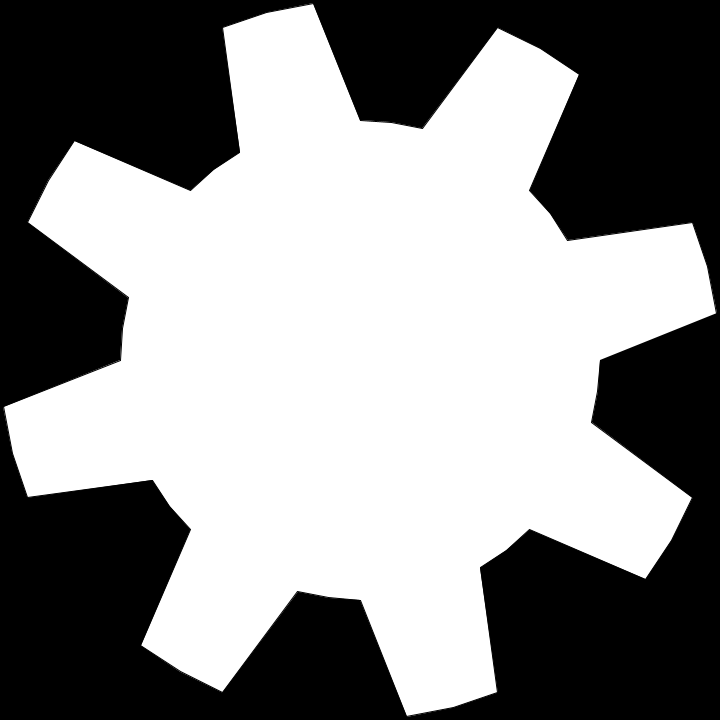
追加説明
半径の大きさのパターンを複数作ることで、複雑な形の歯車を描くことができます。
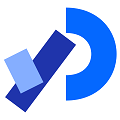
void setup() {
size(720, 720, P2D);
background(0);
int counter = 0;
beginShape();
for (float r = 0; r <TAU; r+= PI / 24) {
float d = 0;
if (counter % 8 >= 6) {
d = 240;
}else if (counter % 8 >= 4) {
d = 360;
}else if (counter % 8 >= 2) {
d = 240;
} else {
d = 120;
}
vertex(cos(r) * d + width / 2, sin(r) * d + height / 2);
counter++;
}
endShape();
}
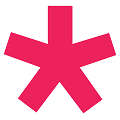
function setup() {
createCanvas(720, 720);
background(0);
var counter = 0;
beginShape();
for (var r = 0; r <TAU; r+= PI / 24) {
var d = 0;
if (counter % 8 >= 6) {
d = 240;
}else if (counter % 8 >= 4) {
d = 360;
}else if (counter % 8 >= 2) {
d = 240;
} else {
d = 120;
}
vertex(cos(r) * d + width / 2, sin(r) * d + height / 2);
counter++;
}
endShape();
}
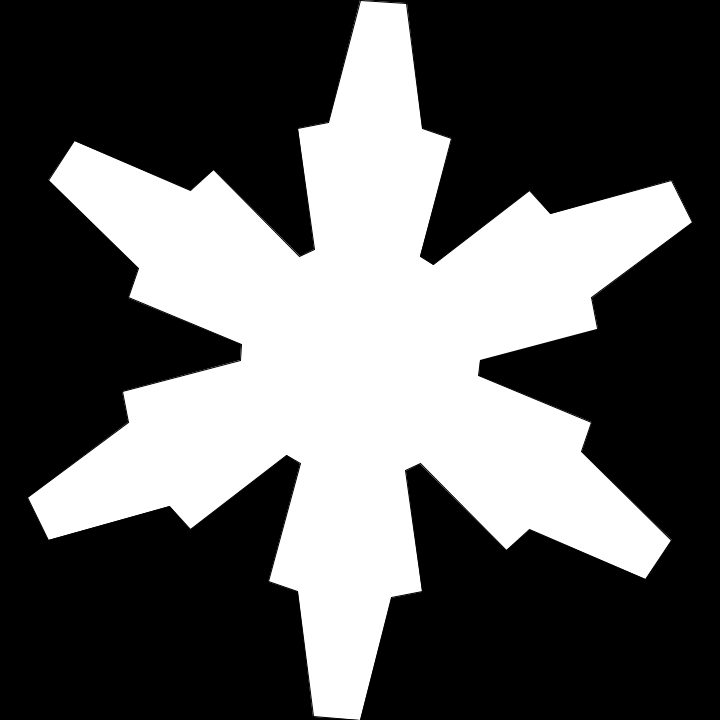
例
型くりこ「Gear/歯車」を使って、二つの歯車をかみ合わせてみた例。
二つの歯車をちょうどよい位置に配置し、それぞれ逆方向で回転させることでかみ合っているような動きになる。
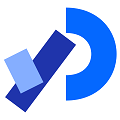
void setup() {
size(720, 720, P2D);
}
void draw() {
background(0);
drawGear(width / 2 - 100, height / 2, 105, frameCount / 100f, 1);
drawGear(width / 2 + 100, height / 2, 105, frameCount / 100f, -1);
}
void drawGear(float x, float y, float length, float angle, int direction) {
int counter = 4 + 1 * direction; // 歯車のずれを作るためdirectionを使う
beginShape();
for (float r = 0; r <TAU; r+= PI / 24) {
float d = 0;
if (counter % 4 >= 2) {
d = length;
} else {
d = length / 4 * 3;
}
vertex(cos((r + angle) * direction) * d + x, sin((r + angle) * direction) * d + y);
counter++;
}
endShape();
}
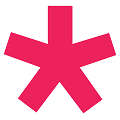
function setup() {
createCanvas(720, 720);
}
function draw() {
background(0);
drawGear(width / 2 - 100, height / 2, 105, frameCount / 100, 1);
drawGear(width / 2 + 100, height / 2, 105, frameCount / 100, -1);
}
function drawGear( x, y, length, angle, direction) {
var counter = 4 + 1 * direction; // 歯車のずれを作るためdirectionを使う
beginShape();
for (var r = 0; r <TAU; r+= PI / 24) {
var d = 0;
if (counter % 4 >= 2) {
d = length;
} else {
d = length / 4 * 3;
}
vertex(cos((r + angle) * direction) * d + x, sin((r + angle) * direction) * d + y);
counter++;
}
endShape();
}
応用編
「三角関数のcos()とsin()を使用して円を描く」方式であるため、同じ方式をとっている下記の「型くりこ Archimedes’ Spiral/アルキメデスの螺旋」と組み合わせることもできます。
型くりこ「Archimedes’ Spiral/アルキメデスの螺旋」と「Gear/歯車」を組み合わせてみた例。
でこぼこの図形が螺旋となって広がっていく。
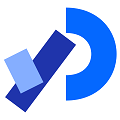
void setup() {
size(720, 720, P2D);
colorMode(HSB, 360, 100, 100, 1);
noStroke();
}
void draw() {
background(0);
int counter = 0;
beginShape(TRIANGLE_STRIP);
for (float r = 0; r <TAU * 11; r+= PI / 24) {
fill((r % TAU) * 57, 100, 100);
float d = 0;
if (counter % 4 >= 2) {
d = r * 9;
} else {
d = r * 9 - TAU * 9 ;
}
float angle = frameCount / 100f;
vertex(cos(r + angle) * d + width / 2, sin(r + angle) * d + height / 2);
counter++;
}
endShape();
}
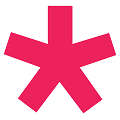
function setup() {
createCanvas(720, 720);
colorMode(HSB);
noStroke();
}
function draw() {
background(0);
var counter = 0;
beginShape(TRIANGLE_STRIP);
for (var r = 0; r <TAU * 11; r+= PI / 24) {
fill((r % TAU) * 57, 100, 100);
var d = 0;
if (counter % 4 >= 2) {
d = r * 9;
} else {
d = r * 9 - TAU * 9 ;
}
var angle = frameCount / 100;
vertex(cos(r + angle) * d + width / 2, sin(r + angle) * d + height / 2);
counter++;
}
endShape();
}
その他作品
型くりこ「Gear/歯車」の考え方を使った作品をいくつか紹介いたします。
#つぶやきProcessing になってしまっているので、コードは圧縮されている箇所が多いですが、基本的な考え方は本記事に記載した内容と同等です。
コメント