型くりこについてはこちらをご覧ください。
目次
「Mirroring Text/鏡文字」について
文字をtext()
で描いた際に、反転した文字も重ねることで元の文字からは想像できないような模様を描く技法です。






ちょっとした新しい記号のような模様が作れるので便利に使うことができます。
使い方
text()
で文字を描く際scale(-1,1)
を使うと文字を左右反転することができます。これを利用して通常の文字と反転した文字を重ねて描きます。
反転する際にはpush()
とpop()
で状態の保存と復元を行うようにし、その中でtranslate()
やscale()
を使って文字の位置などを調整します。
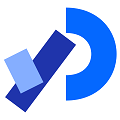
void setup() {
size(720, 720, P2D);
textSize(72);
background(0);
for (int i = 0; i <= 9; i++) {
push();
translate(i*72 + 36, 360);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(-1, 1);
text(i, -10, 0);
pop();
}
}
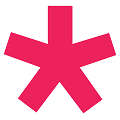
function setup() {
createCanvas(720, 720);
textSize(72);
background(0);
fill(255);
stroke(255);
for (var i = 0; i <= 9; i++) {
push();
translate(i*72 + 36, 360);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(-1, 1);
text(i, -10, 0);
pop();
}
}
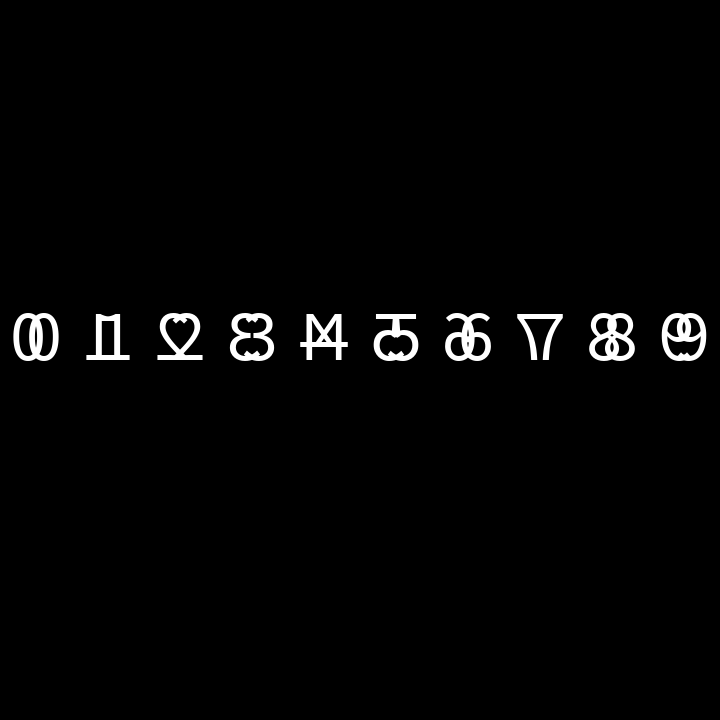
全く新しい記号に見える。
追加説明
scale(1,-1)
で上下反転になります。同様にscale(-1,-1)
で左右上下反転になります。
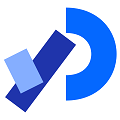
void setup() {
size(720, 720, P2D);
textSize(72);
background(0);
for (int i = 0; i <= 9; i++) {
push();
translate(i * 72 + 36, 360);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(-1, 1);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(1, -1);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(-1, -1);
text(i, -10, 0);
pop();
}
}
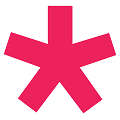
function setup() {
createCanvas(720, 720);
textSize(72);
background(0);
fill(255);
stroke(255);
for (var i = 0; i <= 9; i++) {
push();
translate(i * 72 + 36, 360);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(-1, 1);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(1, -1);
text(i, -10, 0);
pop();
push();
translate(i * 72 + 36, 360);
scale(-1, -1);
text(i, -10, 0);
pop();
}
}
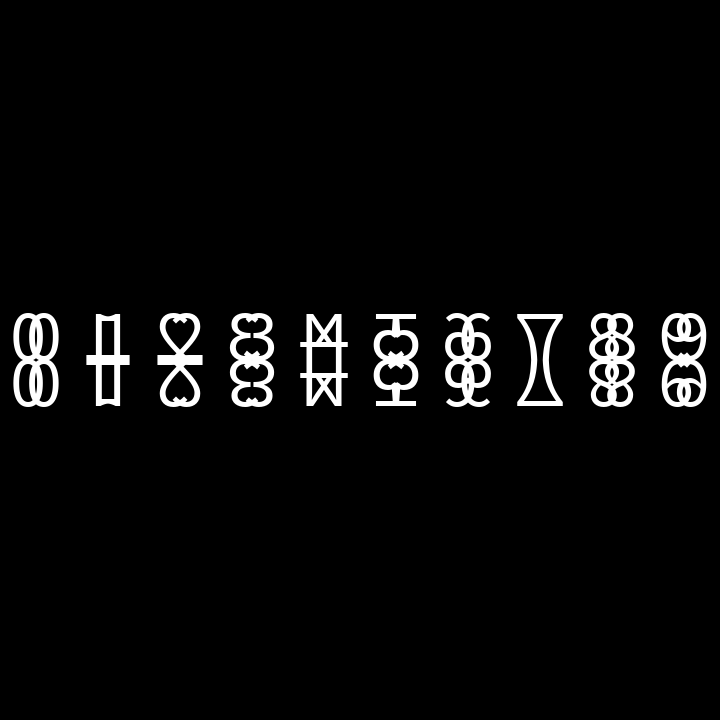
普段見慣れている文字でも、ぱっと見では元の文字を推測するのは難しい。
例
型くりこ「Mirroring Text/鏡文字」を使って、アルファベットを反転させて配置してみた例。
Java版、p5.js版ともそれぞれの方法でrandom()
で0~25の数値を生成し、それを文字に変換することでA~Zの文字を描いています。
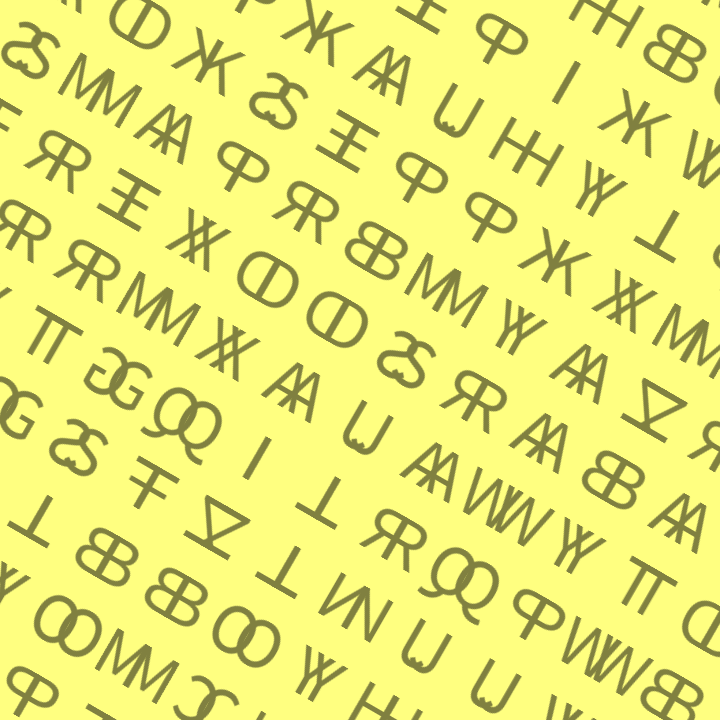
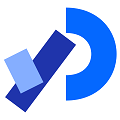
void setup() {
size(720, 720, P2D);
textSize(72);
background(255, 255, 128);
fill(128, 128, 64);
translate(width / 2, height / 2);
rotate(PI / 6);
translate(-width / 2, -height / 2);
for(int y = -height / 2; y <= height * 1.5; y += 80){
for (int x = -width ; x <= width * 1.5; x += 80) {
char c = (char)('A' + int(random(26))); // char型にキャストすればよい
push();
translate(x, y);
text(c, -10, 0);
pop();
push();
translate(x, y);
scale(-1, 1);
text(c , -10, 0);
pop();
}
}
}
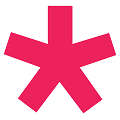
function setup() {
createCanvas(720, 720);
textSize(72);
background(255, 255, 128);
fill(128, 128, 64);
stroke(128, 128, 64);
translate(width / 2, height / 2);
rotate(PI / 6);
translate(-width / 2, -height / 2);
for (var y = -height / 2; y <= height * 1.5; y += 80) {
for (var x = -width; x <= width * 1.5; x += 80) {
var c = char(int(unchar('A') + random(26))); //unchar()で数値にしてchar()で文字に戻せばよい
push();
translate(x, y);
text(c, -10, 0);
pop();
push();
translate(x, y);
scale(-1, 1);
text(c, -10, 0);
pop();
}
}
}
応用編
数字やアルファベット以外の文字も使用することができます。
型くりこ「Mirroring Text/鏡文字」を使って、日本語を反転して重ねてみた例。
Java版の場合は、日本語対応フォントを作成して適用する必要があります。
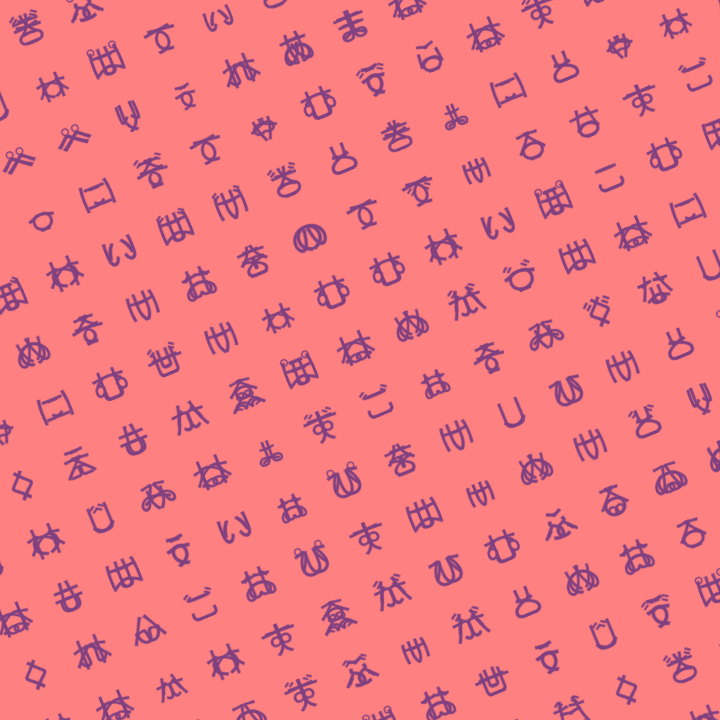
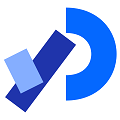
void setup() {
size(720, 720, P2D);
background(255,128, 128);
fill(128, 64, 128);
PFont f = createFont("MS ゴシック", 36); // 日本語対応フォントの生成
textFont(f);
translate(width / 2, height / 2);
rotate(-PI / 8);
translate(-width / 2, -height / 2);
for(int y = -height / 2; y <= height * 1.5; y += 60){
for (int x = -width ; x <= width * 1.5; x += 60) {
char c = (char)('あ' + int(random(85)));
push();
translate(x, y);
text(c, -20, 0);
pop();
push();
translate(x, y);
scale(-1, 1);
text(c , -20, 0);
pop();
}
}
}
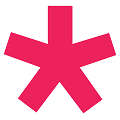
function setup() {
createCanvas(720, 720);
textSize(36);
background(255, 128, 128);
fill(128, 64, 128);
stroke(128, 64, 128);
translate(width / 2, height / 2);
rotate(-PI / 8);
translate(-width / 2, -height / 2);
for (var y = -height / 2; y <= height * 1.5; y += 60) {
for (var x = -width; x <= width * 1.5; x += 60) {
var c = char(int(unchar('あ') + random(85)));
push();
translate(x, y);
text(c, -20, 0);
pop();
push();
translate(x, y);
scale(-1, 1);
text(c, -20, 0);
pop();
}
}
}
その他作品
型くりこ「Mirroring Text/鏡文字」の考え方を使った作品をいくつか紹介いたします。
#つぶやきProcessing になってしまっているので、コードは圧縮されている箇所が多いですが、基本的な考え方は本記事に記載した内容と同等です。
コメント