型くりこについてはこちらをご覧ください。
目次
「Symmetrical Ruler/対象定規」について
図形を、ある線を基準に対称的に配置することで綺麗な模様を描く技法です。






左右対称などでもよいと思いますが、私は特に円型で使うことが多いです。
「対象定規」は一般用語で、お絵描き系のアプリなどで同じような機能が使えたりします。
使い方
いろいろなやり方がありますが、ここでは「時計回りに動く」「反時計回りに動く」を交互に繰り返すことで対称的に図形を動かします。
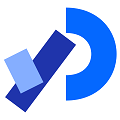
void setup() {
size(720, 720, P2D);
}
void draw() {
background(0);
int direction = 1; // どちらの方向に動くかのフラグ
for (float r = 0; r < TAU; r += PI / 4) {
float angle = r + sin(frameCount / 50f) / 3 * direction;
float x = cos(angle) * 300 + width / 2;
float y = sin(angle) * 300 + height /2;
circle(x, y, 20);
direction = -direction; // 動く方向を反転する
}
}
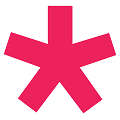
function setup() {
createCanvas(720, 720);
}
function draw() {
background(0);
var direction = 1; // どちらの方向に動くかのフラグ
for (var r = 0; r < TAU; r += PI / 4) {
var angle = r + sin(frameCount / 50) / 3 * direction;
var x = cos(angle) * 300 + width / 2;
var y = sin(angle) * 300 + height /2;
circle(x, y, 20);
direction = -direction; // 動く方向を反転する
}
}
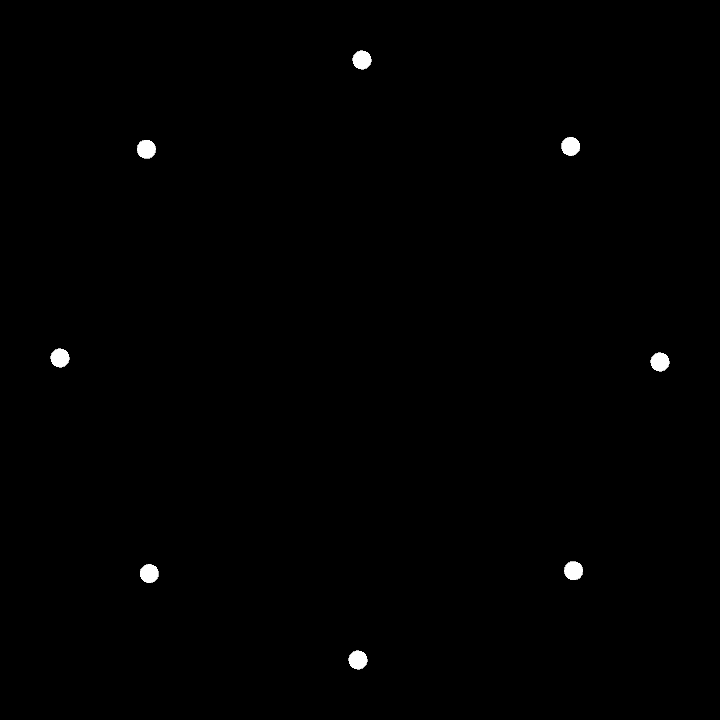
direction
によって、「時計回りに動く」「反時計回りに動く」が交互に現れて対称的な動きになる。追加説明
軌跡を残すようにすると対称性がより見えるようになります。
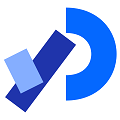
void setup() {
size(720, 720, P2D);
}
void draw() {
int direction = 1;
for (float r = 0; r < TAU; r += PI / 4) {
float angle = r + sin(frameCount / 50f) / 3 * direction;
float length = noise(frameCount / 200f) * 500;
float x = cos(angle) *length + width / 2;
float y = sin(angle) * length + height /2;
circle(x, y, 20);
direction = -direction;
}
}
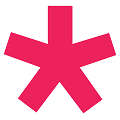
function setup() {
createCanvas(720, 720);
}
function draw() {
var direction = 1;
for (var r = 0; r < TAU; r += PI / 4) {
var angle = r + sin(frameCount / 50) / 3 * direction;
var length = noise(frameCount / 200) * 500;
var x = cos(angle) * length + width / 2;
var y = sin(angle) * length + height /2;
circle(x, y, 20);
direction = -direction;
}
}
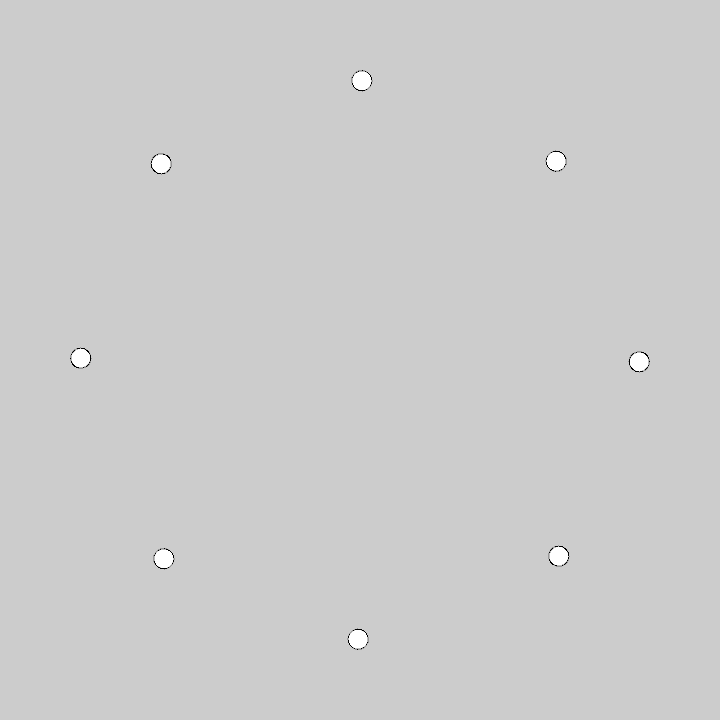
例
型くりこ「Symmetrical Ruler/対称定規」を使って、だんだんと色を変化させる円とその軌跡を描いてみた例。
background(0)
の代わりに薄い黒色を描くことで、軌跡がうっすらと残るようにしています。
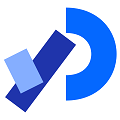
void setup() {
size(720, 720, P2D);
colorMode(HSB, 360, 100, 100, 1);
noStroke();
}
void draw() {
fill(0, 0.02);
rect(0,0, width, height);
fill(frameCount %360, 80, 100);
int direction = 1;
for (float r = 0; r < TAU; r += PI / 8) {
float angle = r + sin(frameCount / 20f) / 3 * direction;
float length = noise(frameCount / 100f) * 500;
float x = cos(angle) * length + width / 2;
float y = sin(angle) * length + height /2;
circle(x, y, 20);
direction = -direction;
}
}
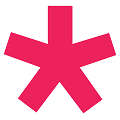
function setup() {
createCanvas(720, 720);
colorMode(HSB);
noStroke();
}
function draw() {
background(0, 0.02);
fill(frameCount %360, 80, 100);
var direction = 1;
for (var r = 0; r < TAU; r += PI / 8) {
var angle = r + sin(frameCount / 20) / 3 * direction;
var length = noise(frameCount / 100) * 500;
var x = cos(angle) * length + width / 2;
var y = sin(angle) * length + height /2;
circle(x, y, 20);
direction = -direction;
}
}
応用編
マウスの座標を使うことで、マウスの動きに合わせて対称定規を使うこともできます。
型くりこ「Symmetrical Ruler/対象定規」で、マウスの座標を使ってみた例。
角度はatan2()
、中心からの距離はdist()
で求めることができる。
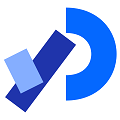
void setup() {
size(720, 720, P2D);
colorMode(HSB, 360, 100, 100, 1);
noStroke();
}
void draw() {
fill(0, 0.02);
rect(0, 0, width, height);
fill(frameCount %360, 80, 100);
int direction = 1;
for (float r = 0; r < TAU; r += PI / 8) {
float angle = atan2(mouseY - height / 2, mouseX - width / 2);
float angleOffset = (PI / 16 - angle) * direction;
float length = dist(mouseX, mouseY, width / 2, height / 2);
float x = cos(r + angleOffset) *length + width / 2;
float y = sin(r + angleOffset) * length + height /2;
circle(x, y, 20);
direction = -direction;
}
}
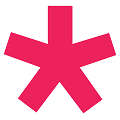
function setup() {
createCanvas(720, 720);
colorMode(HSB);
noStroke();
}
function draw() {
background(0, 0.02);
fill(frameCount %360, 80, 100);
var direction = 1;
for (var r = 0; r < TAU; r += PI / 8) {
var angle = atan2(mouseY - height / 2, mouseX - width / 2);
var angleOffset = (PI / 16 - angle) * direction;
var length = dist(mouseX, mouseY, width / 2, height / 2);
var x = cos(r + angleOffset) *length + width / 2;
var y = sin(r + angleOffset) * length + height /2;
circle(x, y, 20);
direction = -direction;
}
}
その他作品
型くりこ「Symmetrical Ruler/対称定規」の考え方を使った作品をいくつか紹介いたします。
#つぶやきProcessing になってしまっているので、コードは圧縮されている箇所が多いですが、基本的な考え方は本記事に記載した内容と同等です。
コメント